My goal is to implement the physics of different objects and to handle the collision with planes. I first implemented a model for a cube and for a coin.
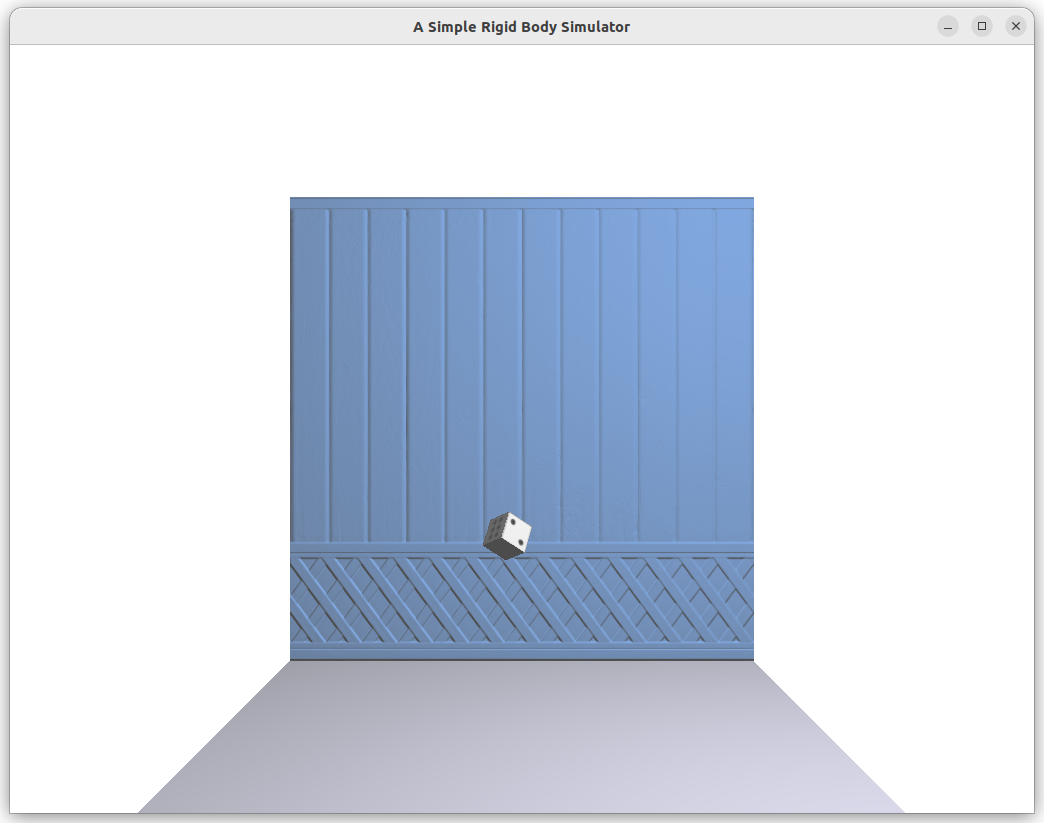
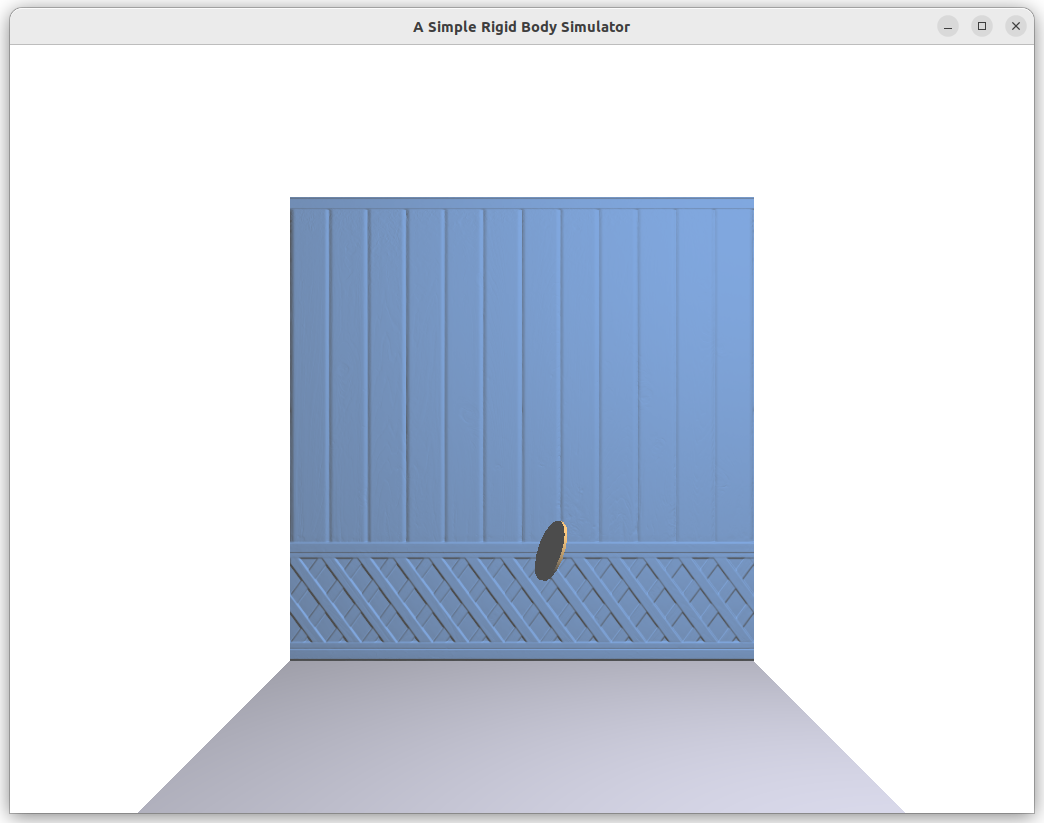
However if we use a rotation matrix, the simulation can quickly give us wrong results, with a cube that grows exponentially.
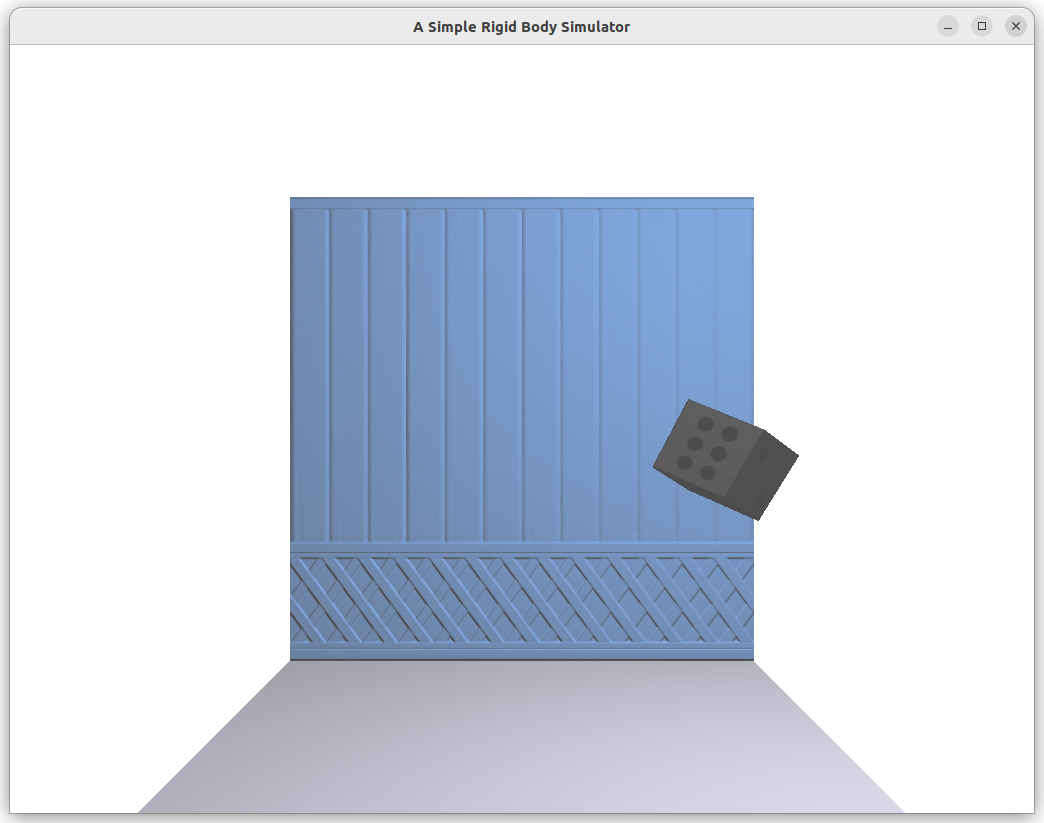
So I implemented quaternions by writing my own class with operators overloading. Now we have a stable simulation.
Then I wanted to implement collisions. At first, I just checked the collision between the box and the floor. To do this, I considered simplifying the floor to be infinite, so that the collision can only occur from above. Then to check the collision, I just needed to find a vertex that is below the floor, and the one in contact is the one at the lowest height.
This approach is interesting with the plane because even if the body completely passes the plane, the collision will still be detected. I then calculate and apply an impulse to the body. However, this cannot determine when the body is out of the floor.
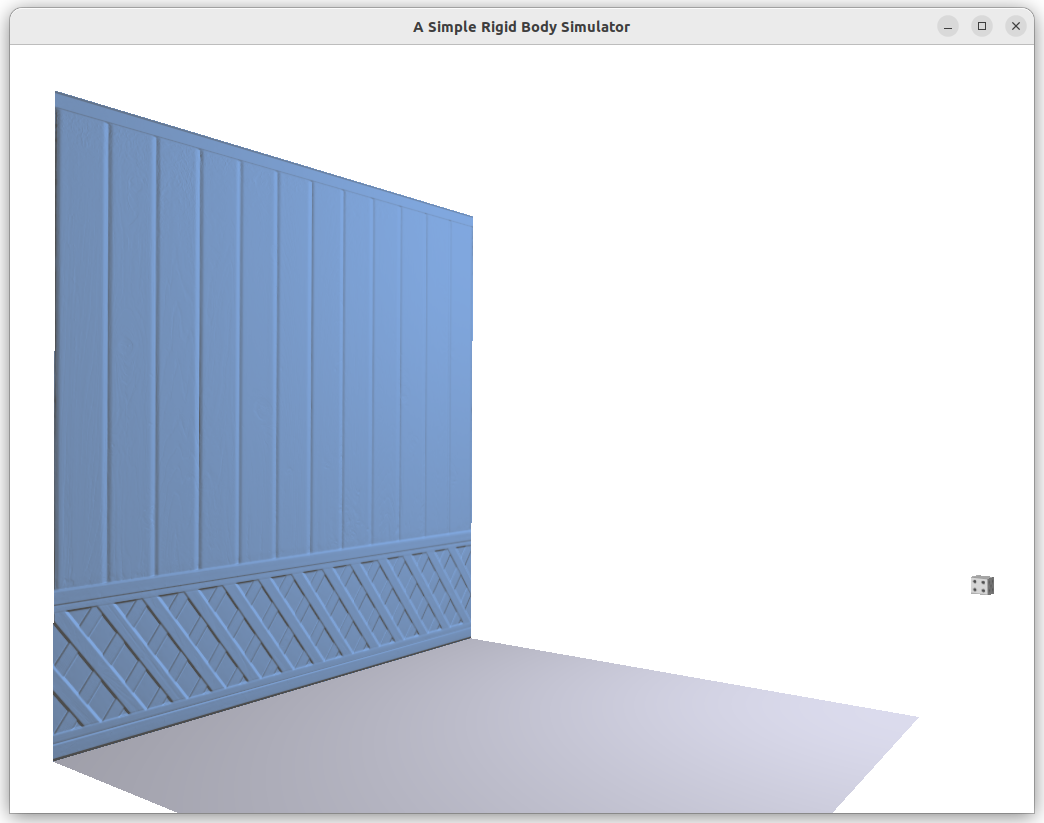
To avoid the bodies going out of the plane, we can multiply P and L by 0.99 at each collision, so that the body will eventually freeze. This can be seen as a friction with the floor.
But this method is not expandable, so we can go further. If we define a normal and a point of the plane, we can project the shape of the box to the normal and see if it is below or above the plane. We can still select the lowest point to be the colliding one. This is in fact a simplified version of the separating axis theorem, applied to infinite planes. With this, we can check collisions with the wall and use slopes.
I then added multiple bodies to the simulation. We can choose the number of cubes and coins by adding arguments when we run the program ./tpRigid N_CUBES N_COINS
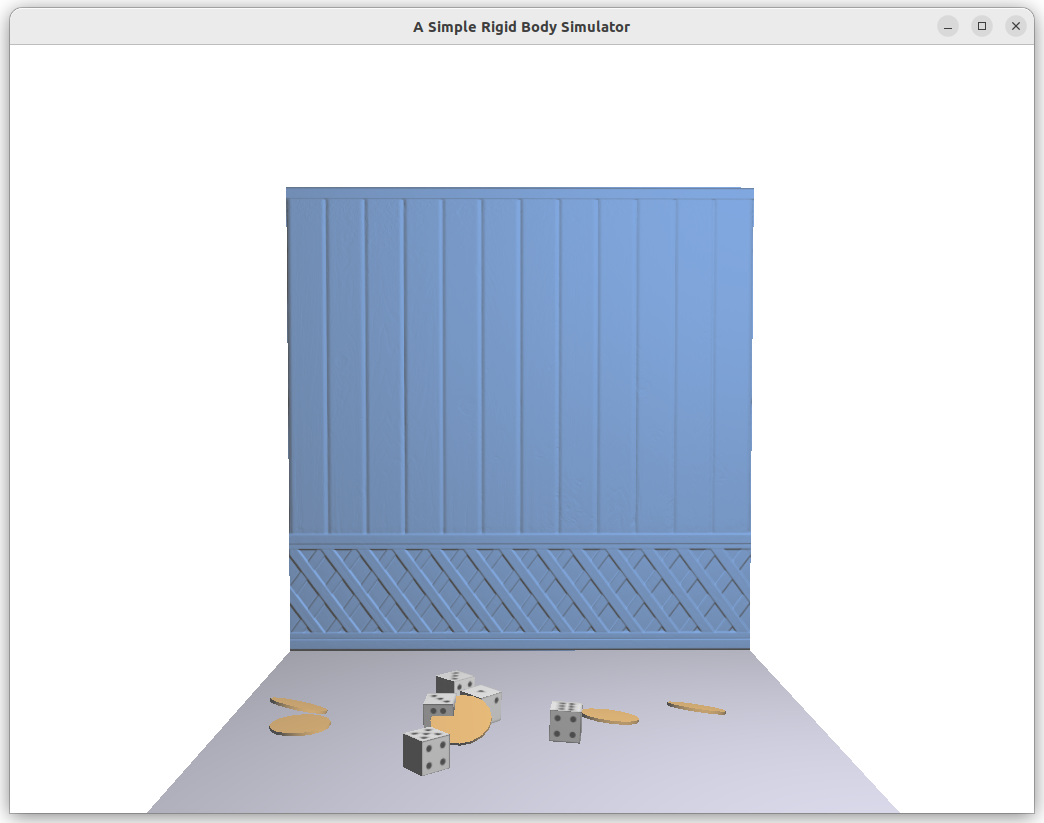