The first thing I did was to display the textures. For the normal mapping, I used the normal from the normal map texture. Normally, I would need to align it to the current vertex normal, but for the wall, the normals are already aligned so I just need to take them.
My first difficulty was with the depthMPV matrix. When I first tried to save the depth buffer, I obtained white images. I took the same projection matrix as the camera, and used lookAt to create my view matrix, and I thought I was doing something wrong. But actually, if we look closely at the image, it is not completely white (the image to the right is more contrasted).
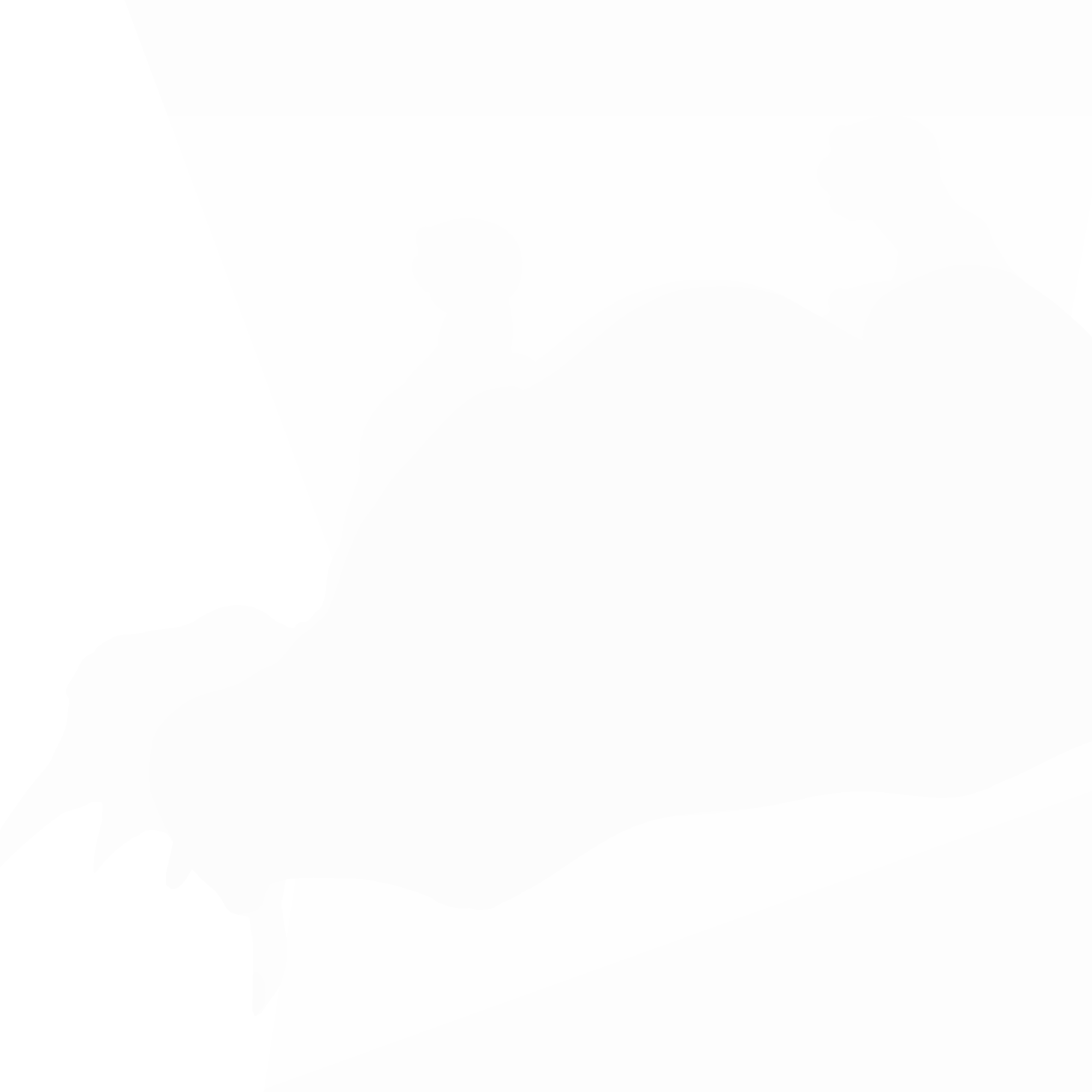
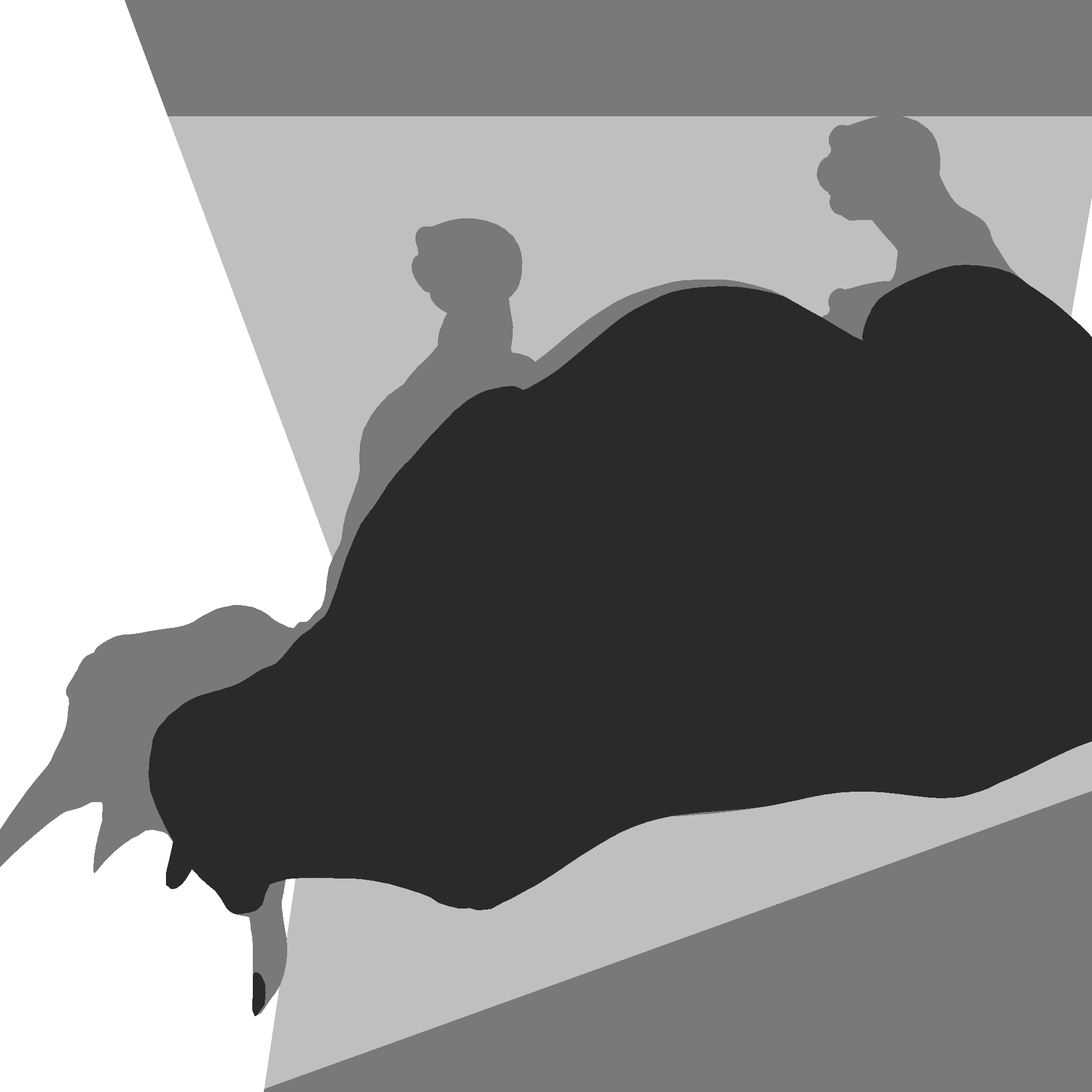
With deeper research, I learned that the z-buffer is not computed linearly with the perspective projection, thus our zone of interest has very close values, that is why we did not see much. To fix this, I still used glm::perspective with the same values as with the camera, but I changed the near plane to be bigger. I then changed the fov to have the whole scene. I wanted to use a perspective matrix rather than an orthonormal because the orthonormal doesn’t take into account the distance of the light, and considers it as infinite. I also saw that I can linearise again the depth if I want to keep a small near plane. But because the lights are not close to the object, it is not needed. Then I obtained those images.
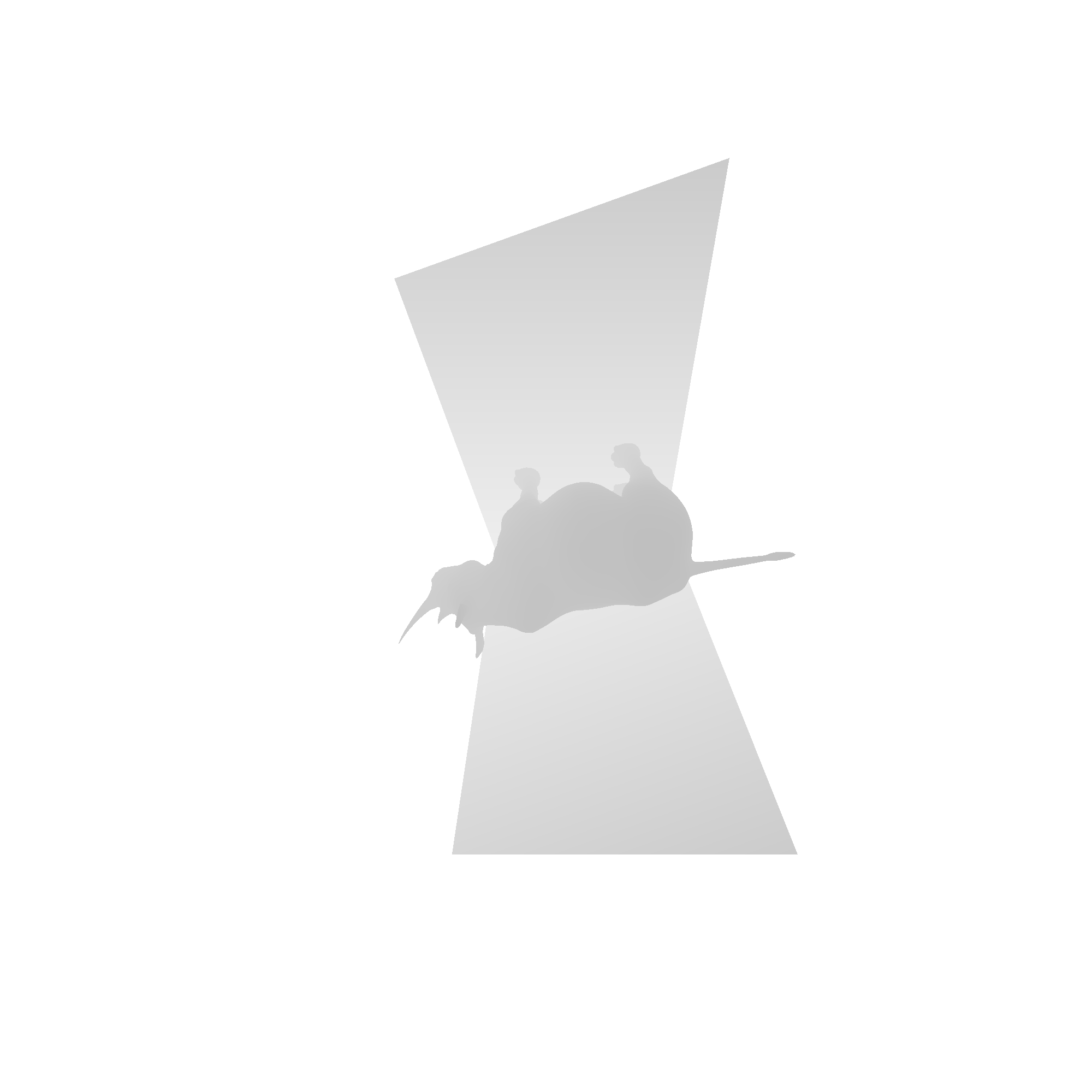
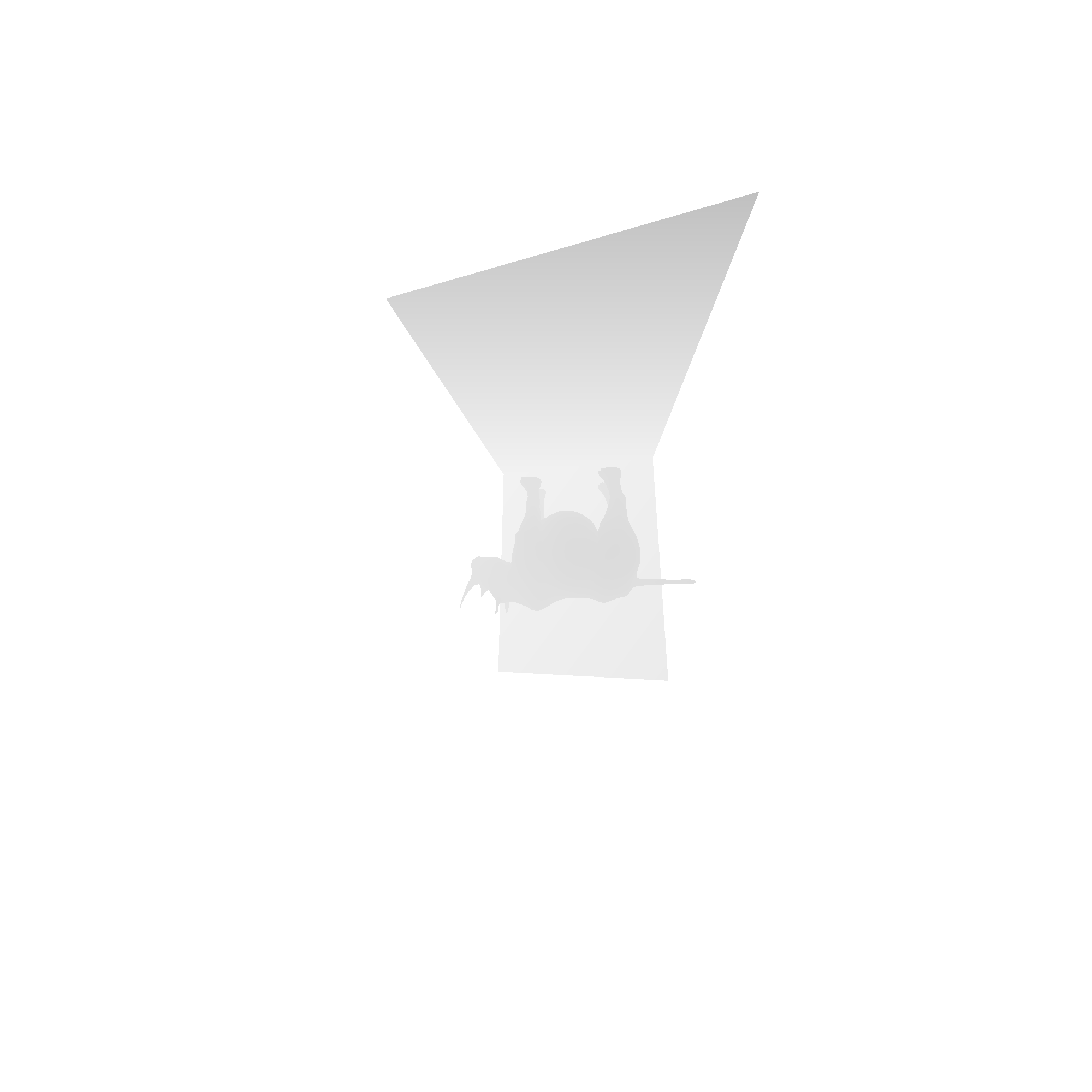
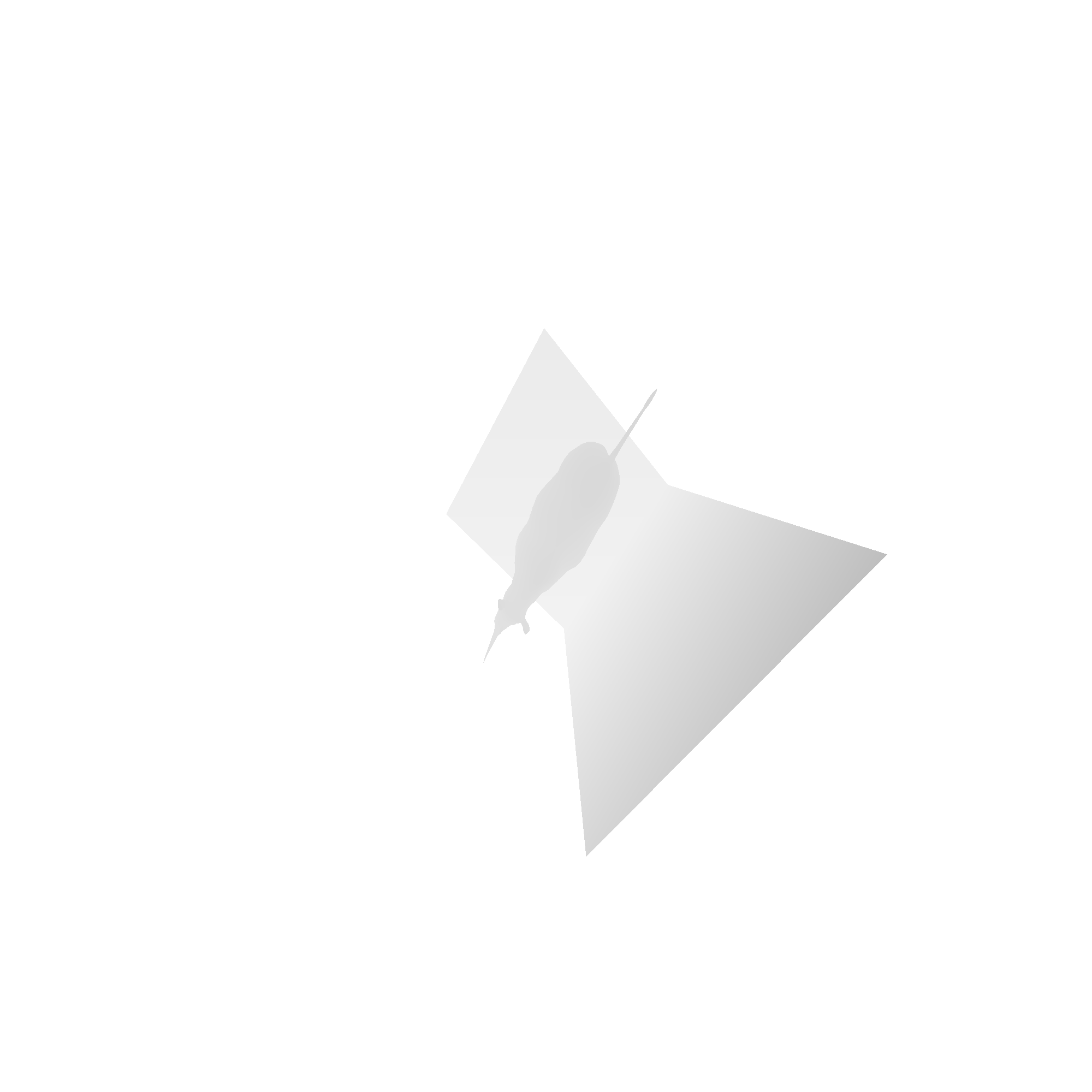
Then, in the fragment shader, I compare the depth of the vertex from the light to the depth map to compute the shadows, but I obtained shadow acne:
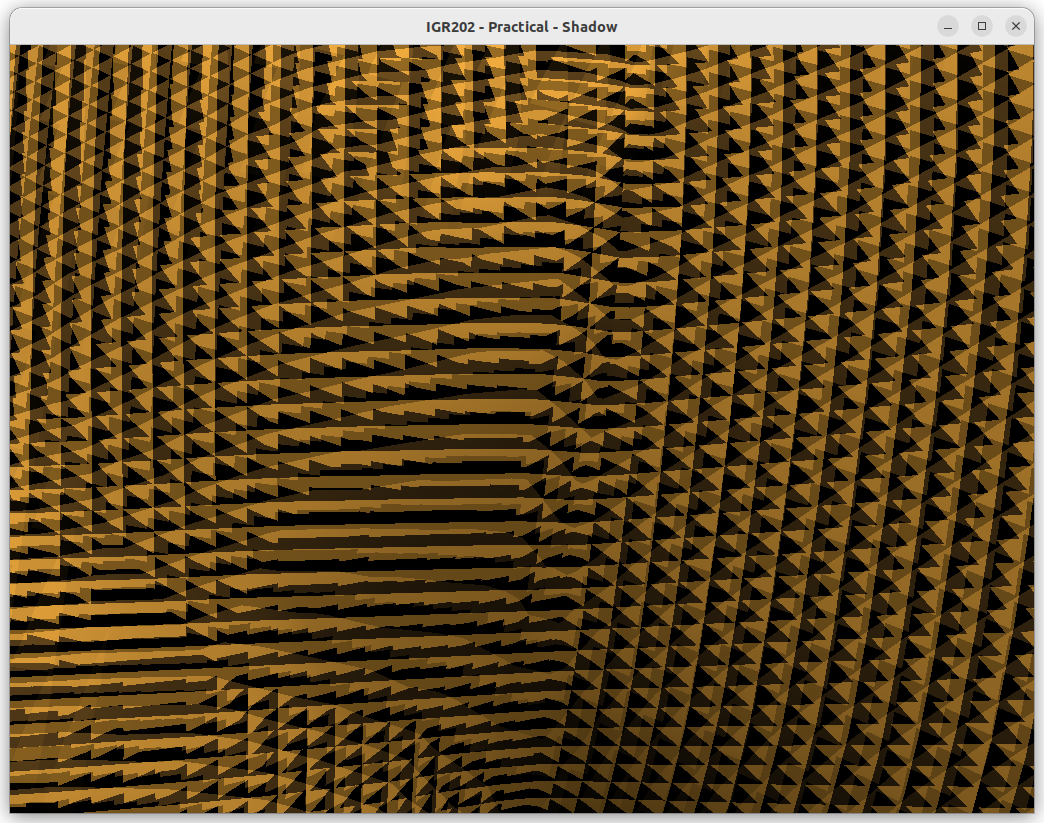
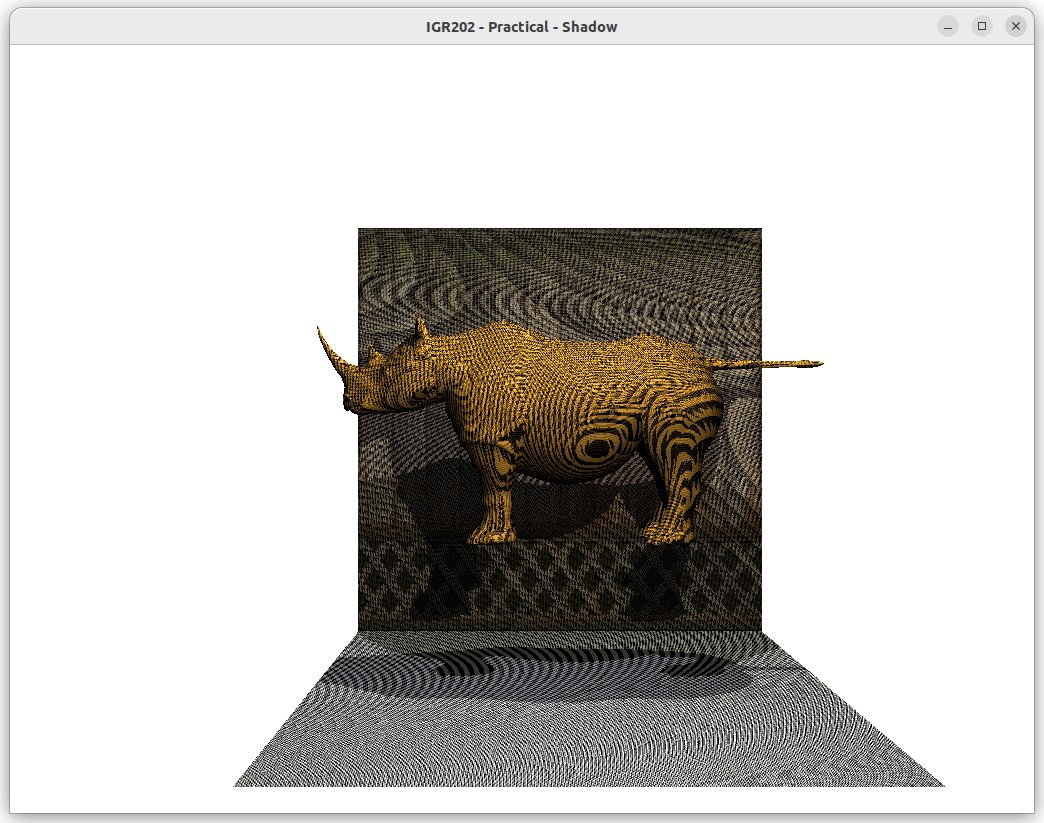
So I included a bias to fix it:
However the shadow was blocky, so I used PCF with a mean filter to smooth it.
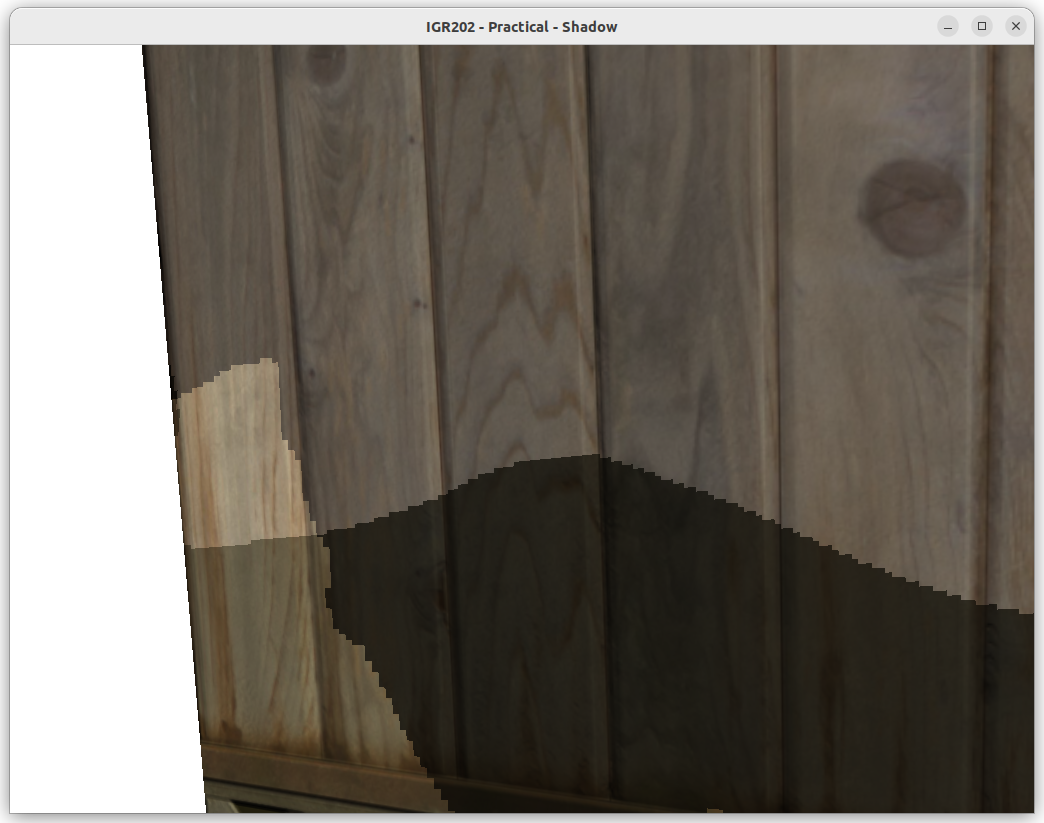
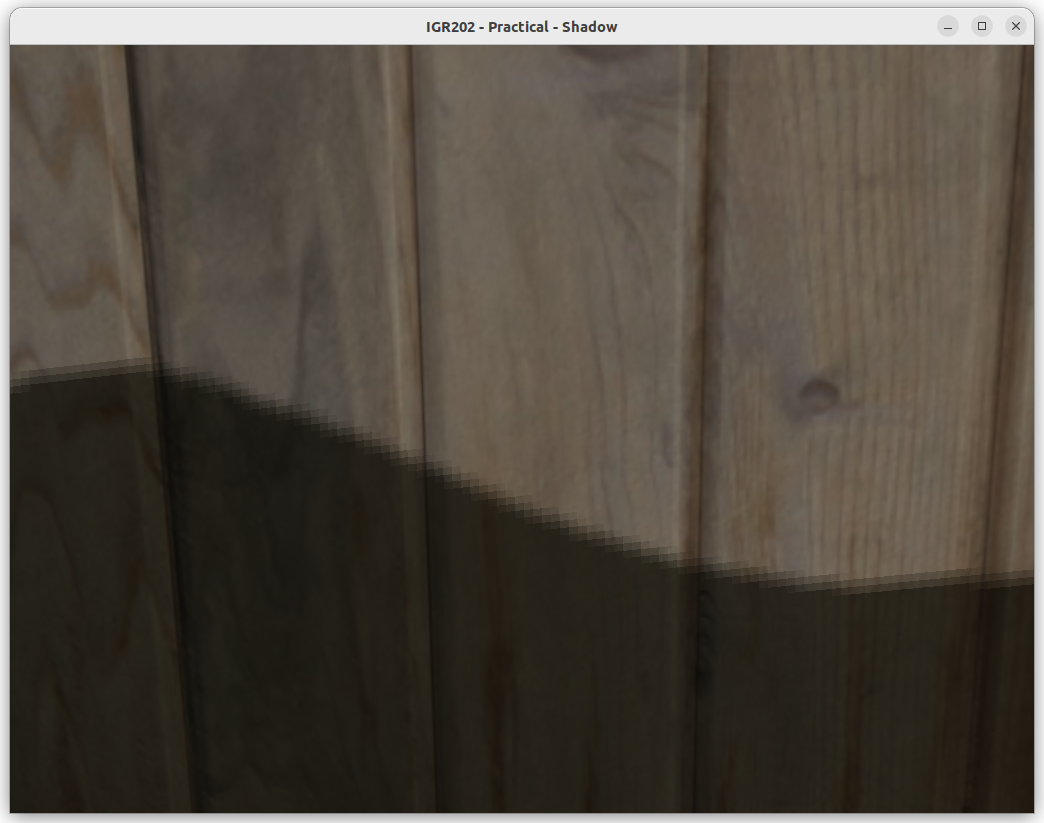
I then noticed that I didn’t need to render the wall and the floor for the shadow mapping, because they are always at the back and will never cause a shadow. So, I removed them in the rendering so I could save computing time and also lower the FOV, to have a closer view on the object and a better definition of the shadow. However, because now the wall is no longer fully in the view of the camera at the position of the light, the texture coordinate could be over 1, so the texture repeats and we have false shadows. We can see it in the first picture on the top left part of the wall. So I first checked in the fragment shader if the texture coordinate is out of image and considered it was not a shadow. Then I saw that I could just tell openGL to clamp the borders:
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_BORDER);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_BORDER);
float borderColor[] = { 1.0f, 1.0f, 1.0f, 1.0f };
glTexParameterfv(GL_TEXTURE_2D, GL_TEXTURE_BORDER_COLOR, borderColor);
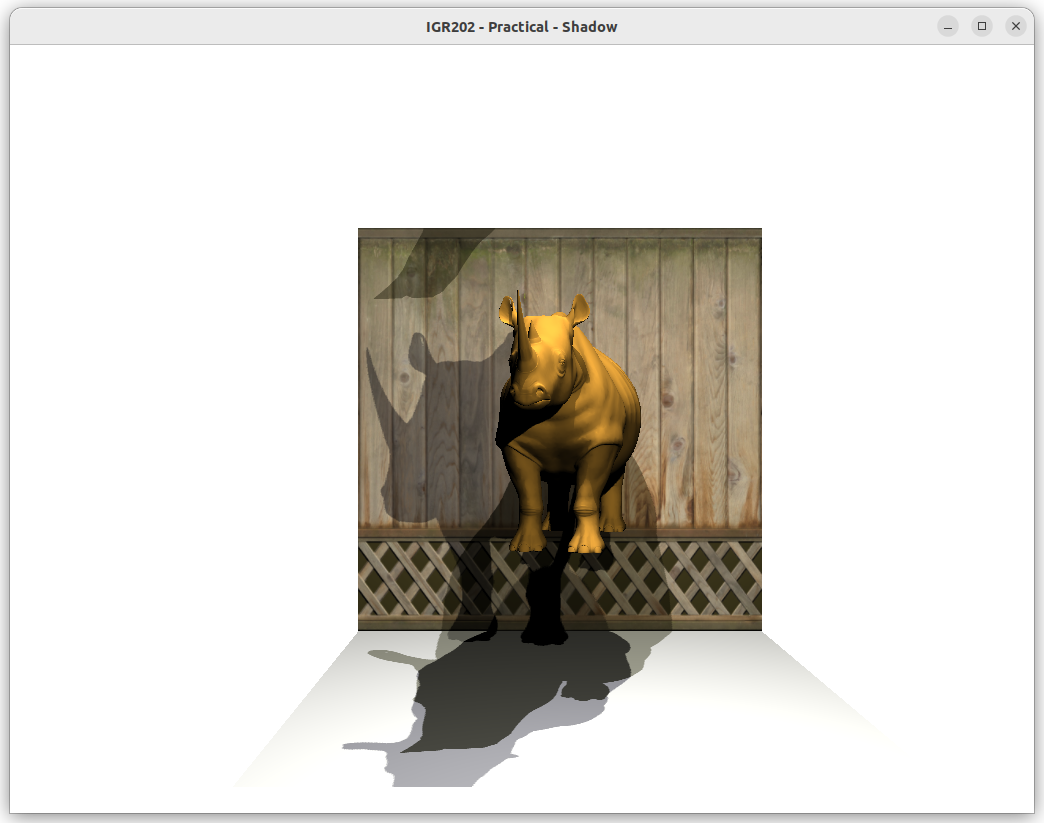
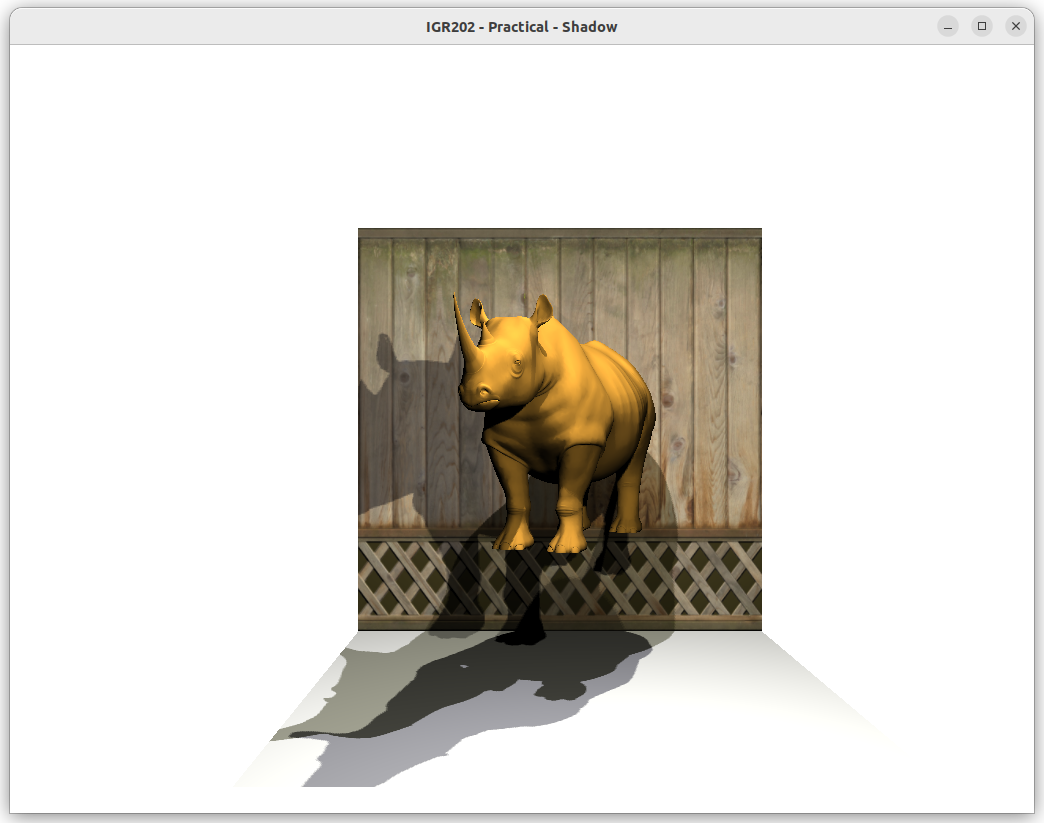