Today I will implement the tutorial from Unity Roll a Ball.
I start by creating a 3D project and add two elements: a plane and a ball. I customize the colors and shinyness to get a pleasing result.
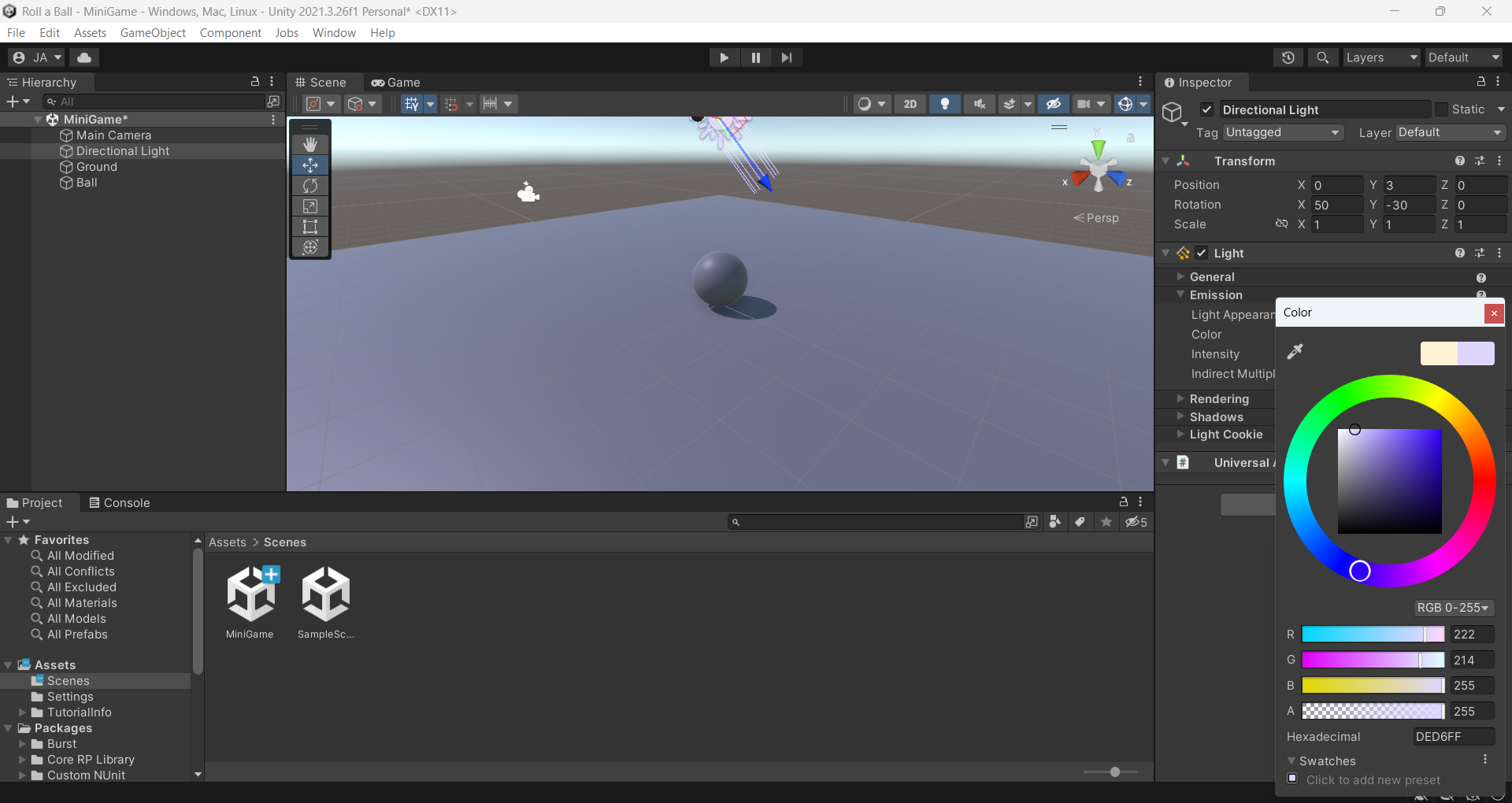
Then I needed to control the ball. To do so, I need to use the Player Input, but I couldn't find it. So I searched on the Internet how to find it. Actually, I needed to install it. Later, I noticed that there were a little notice at the beginning off the tutorial to install it. It was in the Input System package that can be be found in the package manager.
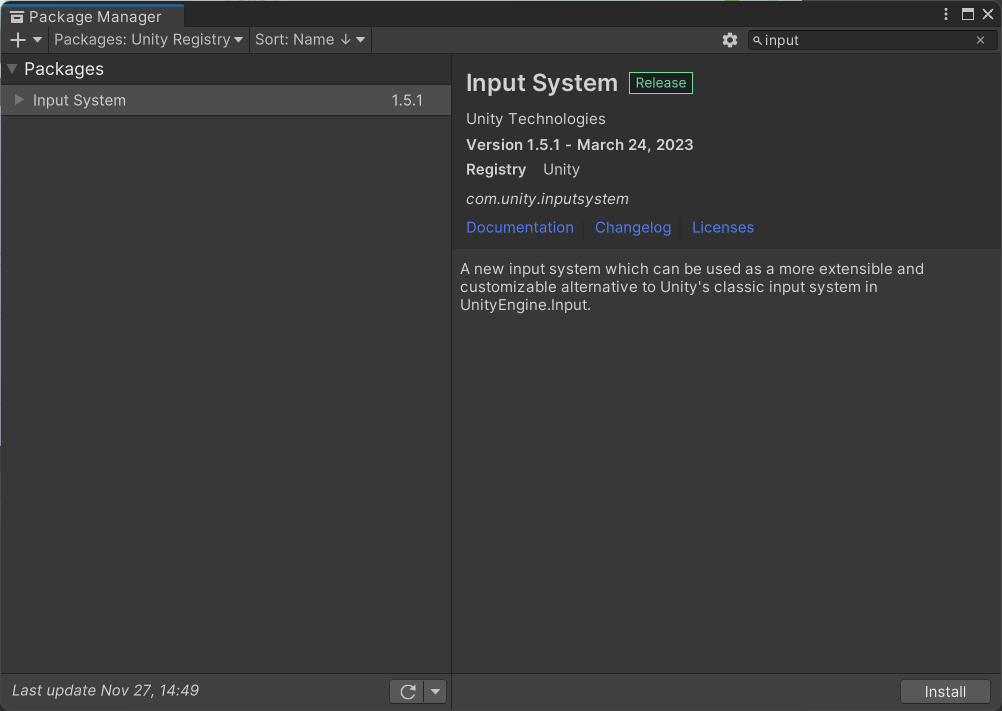
Once the package installed, I added a script to control the ball the keyboard.
Once I had a nice moving ball, I wanted to add a nice effect when the ball moves. I saw when adding a 3D object that we can add a Trail, so that's what I tried. So I created the object and attached it to the player. However the first result was unexpected.
The issue was that the posititon of the trail is relative to its parent. But as the ball rotates, if the trail is not positioned on its center it will rotate too.
But even after fixing this, I wasn't happy with the result. The trail was not looking great, especially on turns, and it was too far away from the ball.
Then I found a video online that has a nice effect. Instead of attaching a 3D object to the player, we can directly add a Trail component to it. It has the same customizations than before but the result is much better. I set the trail to fade out and get thinner with the distance.
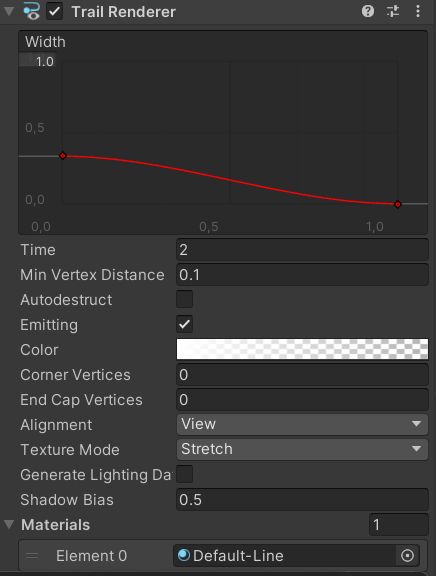
Then I made the camera follow the player. To do this, I attached the camera to the player, but the first result was a fail...
This happened because the camera will rotate with the player when it moves. So to fix this, I need to detach the camera from the player and make a script that updates it position instead. The result is much better.
Then I added collectibles to the scene. Those are little cubes that have a little animation and that dissapear when the player passes on them. The tutorial only showed how to make them spin, but I wanted to make them float a bit too. So I added a translation to the ball with a sinus.
transform.Translate(new Vector3(0, 1, 0) * (float)Math.Sin(Time.time * Math.PI));
However this did not work because the cube is rotating, so its UP vector is changing.
So I change the translation to be relative to the world coordinates by adding the flag Space.World. I also changed the sinus function to its derivative because here we are applying a speed vector to the player.
transform.Translate(new Vector3(0, -(float)(floatRange / Math.PI), 0) * (float)Math.Cos(Time.time * Math.PI), Space.World);
Then this is the final scene with all the collectibles:
The tutorial finished with an AI that is against the player and that is trying to chase it. However, I couldn't implement it because my version of unity (2020) is too old for the AI plugin.